How to URL Encode a String in Golang
2 Mins
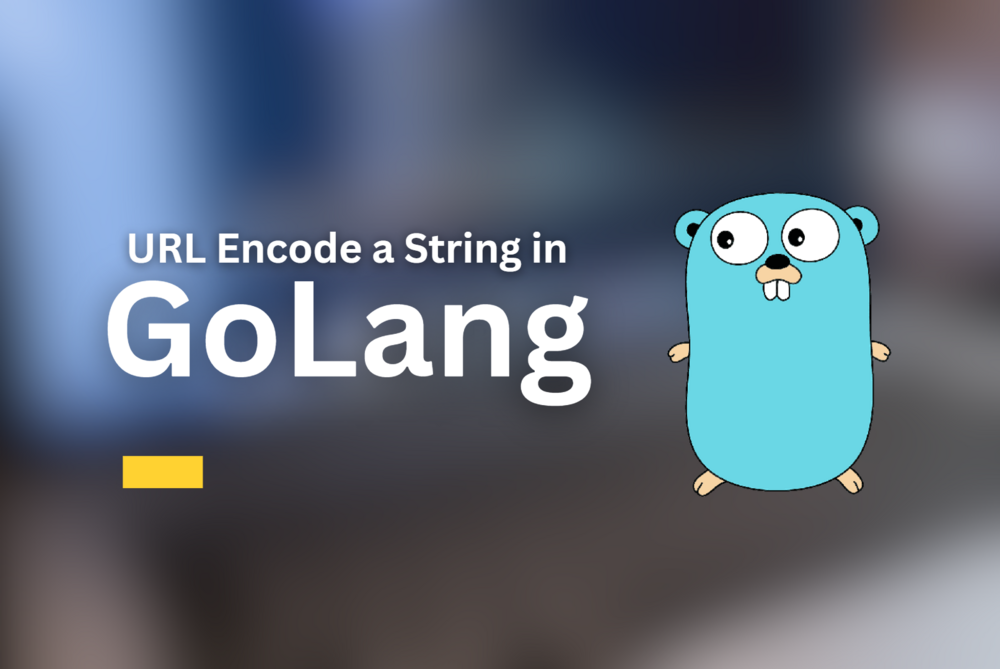
Introduction
URL encoding is a crucial operation in web development to safely include special characters and spaces in URLs. In Go, the net/url
package provides functions for URL encoding. This guide will show you how to URL encode a string in Go, including code examples and detailed explanations.
Code and Explanation
- First, import the
net/url
package to access the URL encoding functions.
import ( "net/url" "fmt" )
- Define the string you want to URL encode.
originalString := "Hello, World! How are you?"
- Use the
url.QueryEscape()
function to URL encode the string. This function replaces spaces and special characters with their respective percent-encoded values.
encodedString := url.QueryEscape(originalString)
- Finally, print the encoded string to see the result.
fmt.Println("Encoded URL:", encodedString)
Full Code Example:
Here's the complete Go code for URL encoding a string:
package main import ( "net/url" "fmt" ) func main() { originalString := "Hello, World! How are you?" encodedString := url.QueryEscape(originalString) fmt.Println("Encoded URL:", encodedString) }
Explanation:
- We import the
net/url
package to access theurl.QueryEscape()
function. - We define the
originalString
that we want to encode. - Using
url.QueryEscape()
, we encode theoriginalString
and store the result inencodedString
. - Finally, we print the
encodedString
, which now contains the URL-encoded version of the original string.
By following these steps, you can safely URL encode strings in Go, ensuring proper data transmission in web applications.